This guide gives a recommended order and basic guidelines for implementing the components and endpoints necessary for Bolt Ignite. Please see the Components, Endpoints, and Styling sections for more information on each of those elements.
Enhance Login and Registration Flows
Step 1: Install the Bolt Embed Script
Before you can use Ignite, install the Bolt Embed Script to every page where a shopper can checkout or access their account. This can include:
- Your login page
- Your account registration page
- Your forgot password pages
- All cart pages
- All checkout pages
- Your order confirmation page
- Your order failed page
Script Options
<script
id="bolt-embed"
type="module"
src="{BASE_URL}/embed.module.js"
data-publishable-key="{your-bolt-publishable-key}"
async
></script>
<script
id="bolt-embed"
type="text/javascript"
src="{BASE_URL}/embed.js"
data-publishable-key="{your-bolt-publishable-key}"
async
></script>
<script
id="bolt-embed"
type="text/javascript"
src="{BASE_URL}/embed.js"
data-publishable-key="{your-bolt-publishable-key}"
></script>
Base URL
Publishable Key
Initialize the Bolt Client
On each page, the Bolt client must be initialized before using any components.
if (Bolt != null) {
initBolt()
} else {
// Use `document.head` if the Bolt script is located in the document <head></head>
// make sure you add the id "bolt-embed" to your Bolt script tag.
document.body.querySelector("#bolt-embed")
.addEventListener("load", initBolt, { once: true });
}
function initBolt() {
const boltPublishableKey = YOUR_KEY;
Bolt.initialize(boltPublishableKey);
// start using Bolt
}
Because you’re loading the Bolt ignite script as an external package, the timing of when the script loads and the execution of your scripts is hard to predict. So we check see the Bolt
global client object exists first. Otherwise, we wait for the script to load before usage.
Step 2: Add the Bolt Login Modal
The Login Modal is how Bolt authenticates shoppers and handles account lookup as well as creation. This is triggered by our Automatic Email Detection.
This should be added anywhere a shopper enters their email address on your site. This is likely: account login page, account registration page, forgot or reset password page, checkout page.
About
The Login modal is a required component that validates and authenticates the shopper before or during the checkout process.
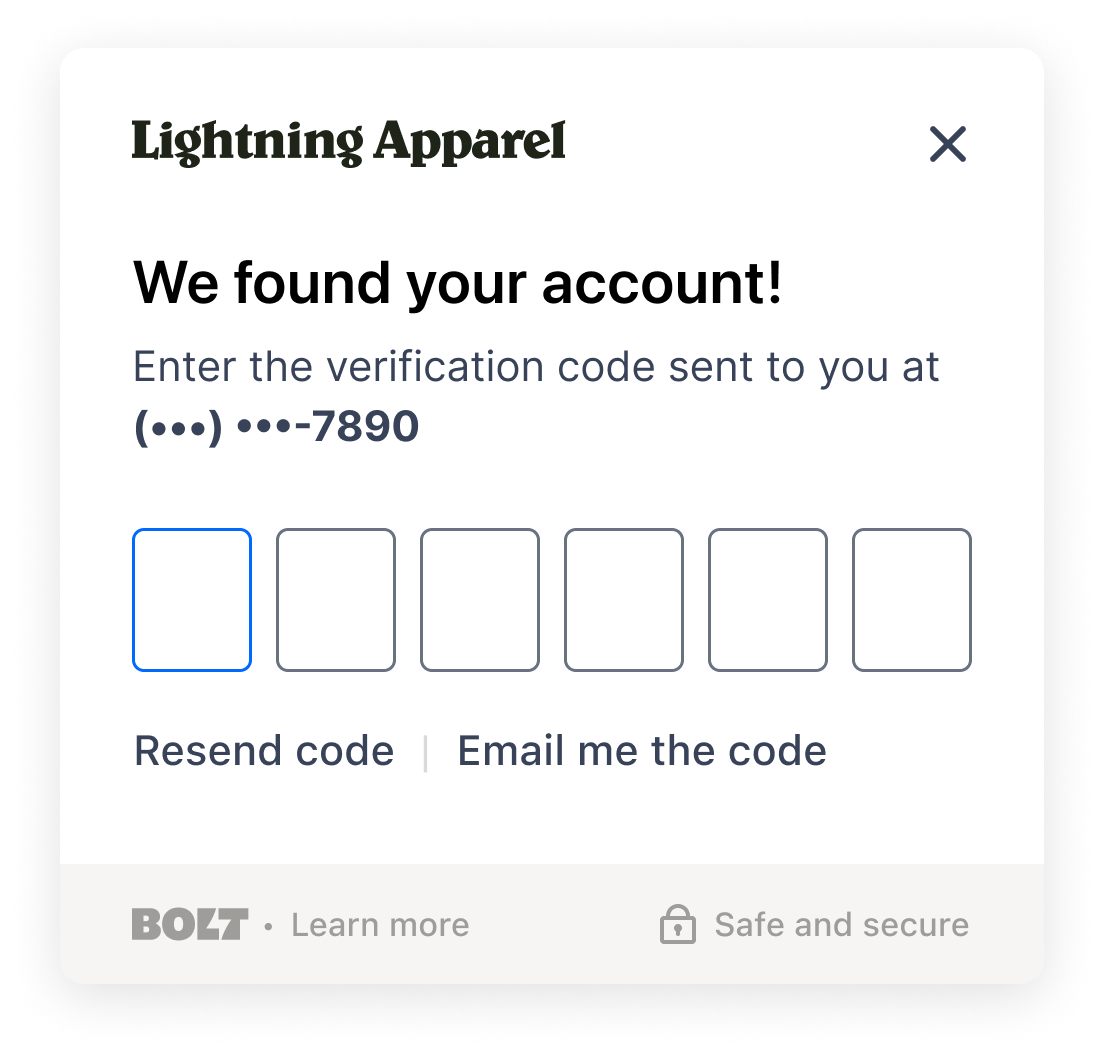
Placement
This component should be attached to all email fields, and it will automatically detect emails typed into the input field. This is called Automatic Email Detection. If the email is recognized as a Bolt Account, they are prompted to input a one-time verification code (OTP) into the Login Modal or use a Passkey. They may also be prompted to complete any missing information.
Implementation
Start by initializing Bolt with your publishable key.
Create the login modal and attach it to your email field.
<script>
async function initBolt() {
const loginModal = Bolt.create("login_modal");
await loginModal.attach("#email-form-group", { context: "checkout" });
Bolt.on("login_succeeded", (loginSuccessResponse) => {
// Use the results to call the Bolt OAuth endpoint in your backend
});
Bolt.on("login_failed", (loginFailResponse) => {
// Error means the user either dismissed the modal, or there was an error
});
}
</script>
<div id="email-form-group">
<input type="email" />
</div>
interface LoginSuccessResponse {
email: string;
result: {
authorizationCode: string;
scope: string;
state?: string;
}
}
interface LoginFailResponse {
email: string;
result: Error;
}
The Login modal will automatically pop up when a Bolt-recognized email is entered. When the proper credentials is entered, login will be completed and the event login_succeeded
and login_complete
(success state) will be fired.
The authorization code received from the Login Modal will enable your store to receive the necessary OAuth access tokens. These OAuth tokens will provide your store access to Bolt Account APIs. To enable your store to access Shopper Account data, see the OAuth reference for more information.
The store frontend should send the authorization code to your store backend and exchange the authorization code for the appropriate OAuth tokens using the OAuth Token endpoint.
Take careful note of handling successful authorization. You should only use authorization results once.
Implement Passwordless Forgot Password Flows
Add the Login Modal to your Forgot Password flow to save shoppers the hassle of recovering or resetting their password.
If a shopper forgets their password, your site might have a “Forgot Password” button to direct them to reset their password. Improve this experience by allowing them a chance to do passwordless login instead.
Placement
Attach this to your forgot password button in all of your sign in screens.
Implementation
<script>
async function initLogin(customerEmail) {
// Same code as above...
Bolt.on("forgot_password_continue", ({ context }) => {
// Proceed to your normal Forgot Password flow
});
}
</script>
<div id="email-form-group">
<input type="email" />
<button type="button" id="forgot-password">Forgot password</button>
</div>
If the shopper completes the Passwordless Forgot Password flow, a login_success
event will fire. Otherwise, listen to forgot_password_continue
event to proceed to reset password the shopper’s password as normal.
Additionally, if your shopper enters their passowrd incorrectly more than once, launch the login_modal
to allow them to access their account without resetting or recovering their password.
Step 3: Add the Bolt Login Button
The Bolt Login Button is used to offer shoppers a quick and passwordless signin experience at multiple points during the shopping journey, including during account registration, account login, and checkout. Different variations of this button will appear depending on the login context
.
On Sign In and Account Registration Pages
The login button will automatically mount below email fields that you have attached the Login Modal to if you used the Login modal with the default settings (autoDetectEmail
is enabled by default).
Alongside Checkout Steps
The Autofill button is a variation of the Login button that allows the shopper to launch the Login modal at any point in checkout (dependent on your placement of the button).
INFO
Even when mounted, the Autofill Button button only appears if a shopper is recognized, but they declined to sign in at an earlier step.
Placement
Mount this component to an element in the top-right corner of checkout steps, specifically after the Payment step.
Implementation
To enable, call mountPasswordlessLoginButton
whith the shopper email and a query selector target, which specifies where to mount the button.
await Bolt.getComponent("login_modal").mountPasswordlessLoginButton(
shopperEmail,
"#autofill-button-container",
"checkout"
);
Step 4: Add the Bolt Signed in Status Banner
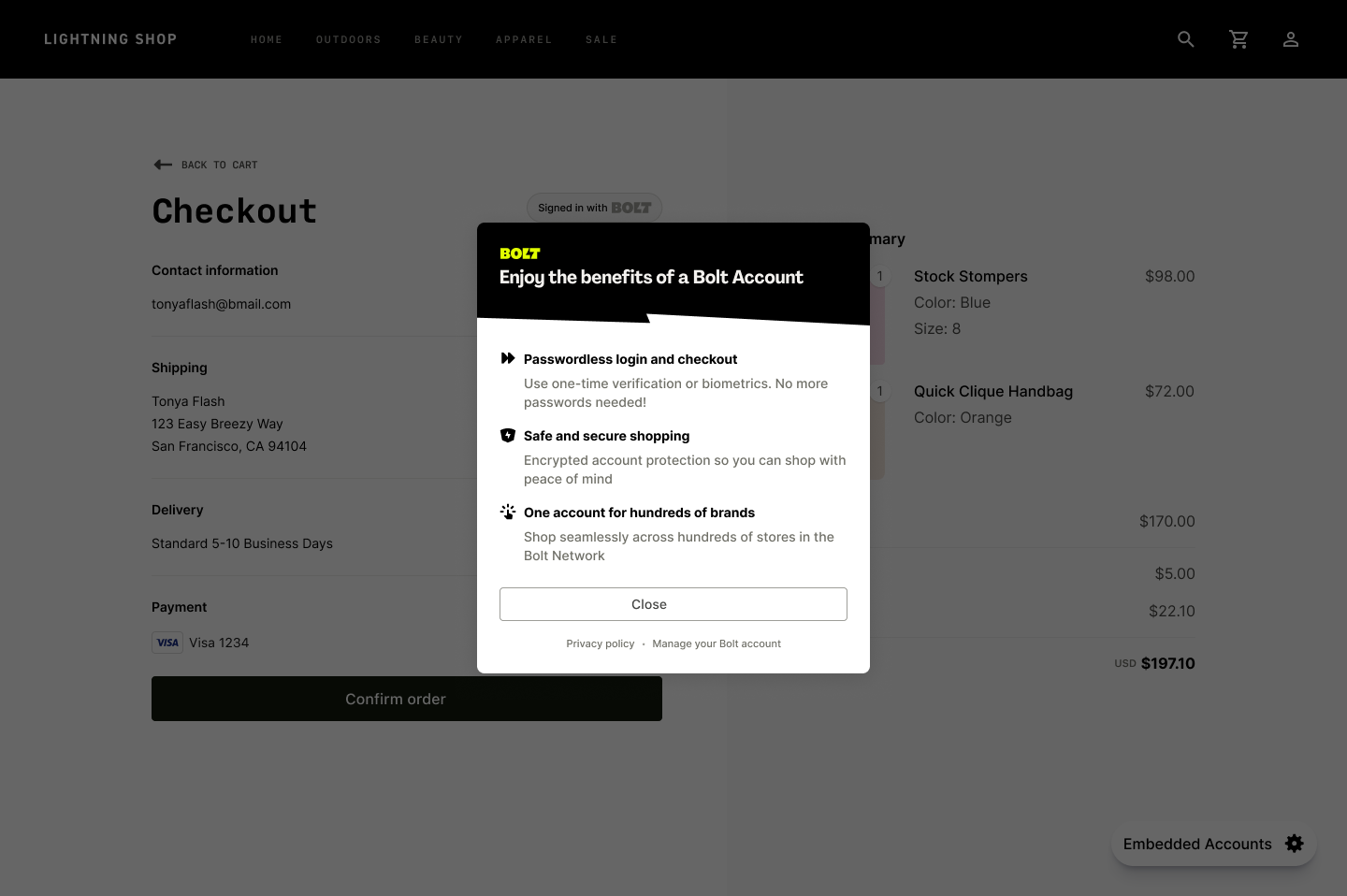
About
The “Signed in with Bolt” button indicates to the shopper that they have successfully signed into your store and Bolt via their credentials and may now enjoy the benefits of their accounts. When clicked, this banner will display information about having a Bolt account.
Placement
Place this button near the top of your Checkout pages to indicate to the shopper they are signed in with Bolt.
Implementation
const loginStatusComponent = Bolt.create("logged_in_with_bolt");
await loginStatusComponent.mount("#logged-in-with-bolt-container");
Step 5: Implement API Requirements
To ensure seamless communication between your store and Bolt, there are 4 APIs to build and modify.
A successful implementation of Bolt Ignite integrates two categories of API requirements. These types of APIs work together to facilitate seamless communication between Bolt and your store.
- Merchant API requirements: Merchant-hosted endpoints Bolt calls get and update shopper data within your system.
- Bolt API Requirements: Bolt-hosted endpoints that your store will use to get and update data from Bolt.
Implement the Merchant Callback APIs
The Merchant Callback API is a custom implementation that you must build on your cart platform, that Bolt will send requests to in relation to as part of the shopper lifecycle.
Build the Get Account Endpoint
Build the Upsert Account Endpoint
Call Bolt Login APIs
We’ll only highlight the most important API endpoints to get you running, but you should refer to our full API documentation and endpoints guide for more information.
Build the OAuth Login Endpoint
De-duplicate Account Information in Checkout
Build the Authorize Endpoint
Configure Shipping Restrictions (optional)
Enhance Payments
Step 6: Add the Bolt Payment Field Component
Use our customizable Payment Fields for Bolt’s out-of-the-box credit card tokenization.
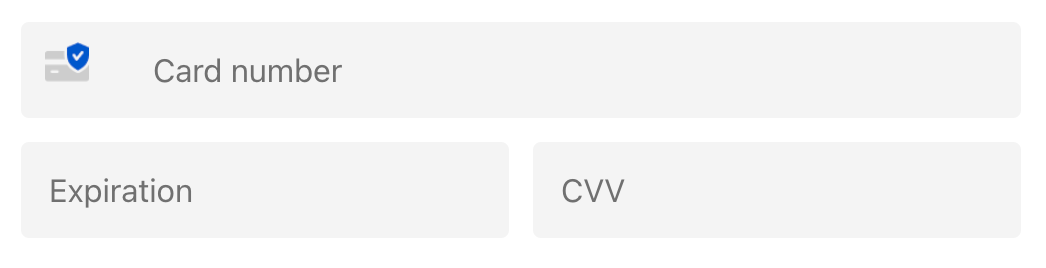
About
Add payment fields to your store’s front end to leverage Bolt’s out-of-the-box credit card tokenization and enable Bolt account creation. Follow our Component Style Guide to completely customize this component’s inputs to match your site.
Implementation
Mount the payment fields component at the payment step of your checkout:
<script>
const paymentComponent = Bolt.create("credit_card_input");
paymentComponent.mount("#credit-card-fields");
document.querySelect("#submit-payment").addEventListener("click", () => {
const tokenize = await paymentComponent.tokenize();
// store these results for the final authorize payment
});
</script>
<section id="payment-section">
<div id="credit-card-fields"></div>
<button id="submit-payment">Continue</button>
</section>
Then, call paymentComponent.tokenize()
when you are ready to retrieve a token for the entered payment information. It contains all information necessary to call the Authorize Payment API as its parameter.
Step 7: Add the Account Creation Checkbox
Add Bolt’s Account Creation Checkbox to the payment step of your checkout process to increase account creation back to your customer database and Bolt’s Shopper Network; this ensures that your shoppers gain access to Bolt’s One-Click Checkout on subsequent visits to your store.
About
When a shopper is not using one-click checkout and does not have a Bolt account, you should render Bolt’s account creation checkbox to collect their consent to create a Bolt account and store account, if applicable. If they accept, this enables a one-click checkout experience the next time they return to your store.
Shopper Experience
During checkout, shoppers without a pre-existing Bolt account have the option to opt out of creating one. Bolt provides an embedded component for collecting consent and displaying terms and conditions.
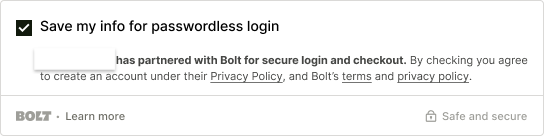
Implementation
Mount the checkbox at your payment or review step of your checkout.
const shouldCreateBoltAccount = true;
const accountCheckboxComponent = Bolt.create("account_checkbox", {
merchantName: "ABC Store",
defaultValue: shouldCreateBoltAccount,
});
accountCheckboxComponent.mount("#payment-section");
accountCheckboxComponent.on(
"change",
(checked) => (shouldCreateBoltAccount = checked)
);
You can build logic to determine the visibility of the checkbox based on whether the shopper is logged into their Bolt account as well as whether their email address is already associated with a Bolt account. For example:
const shopperEmail = ""; // This is the email the shopper entered during checkout
let hasBoltAccount = false;
let isSignedIntoBolt = false;
Bolt.on("account_check_complete", ({email, result}) => {
hasBoltAccount = email === shopperEmail && result;
}, { replayLast: true });
Bolt.on("login_succeeded", ({email}) => {
isSignedIntoBolt = email === shopperEmail;
}, { replayLast: true });
Bolt.on("logout", () => {
isSignedIntoBolt = false;
});
function onPaymentStep() {
if (!hasBoltAccount || !isSignedIntoBolt) {
accountCheckboxComponent.mount("#payment-section");
}
}
When calling Bolt’s Authorize Payment API, pass the value in create_bolt_account
as part of the request object.
In the sample code, adding { replayLast: true }
allows you to get results from the last login event